Suppose our project looks like the one on the right and we found a bug we didn't know existed or what caused it. We could check every single commit one-by-one until we find it, but that is time-consuming. bisect is a Git tool for finding bugs in our code.
In a nutshell, it works by doing a binary search throughout our repository. We'll tell Git of a good commit, and a bad commit and Git will help us zero-in the commit that caused the bug so that we can fix it.
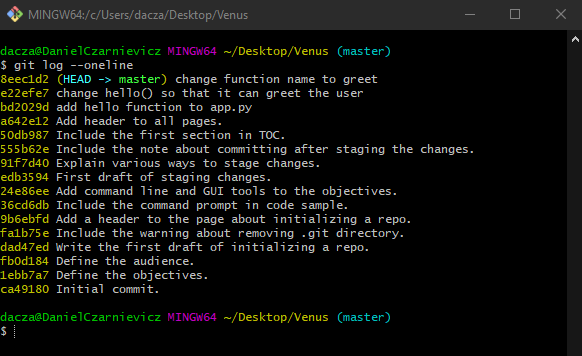
So, imagine 8eec1d2 (our HEAD) is a bad commit (we found a bug here), and ca49180 (our initial commit) will be our first good commit (a commit that we know did not have the issue).
To start bisect we simply run
git bisect start
Notice that Git Bash changed from (master) to (master | BISECTING). Right now our HEAD is at the bad commit (the last one), so we need to tell Git that this is a bad commit. To do that, we run
git bisect bad
Now we need to give Git a good commit. If you didn't write down the hash for a good commit before starting the bisect process don't worry, you can run git log --oneline and get it now. So we run
git bisect good ca49180
Now Git is telling us that we have 7 revisions left (7 commits to check instead of the total 16), and it's also telling us that we can get it done in 3 steps. Notice that Git Bash changed again and is not at commit 24e86ee anymore.
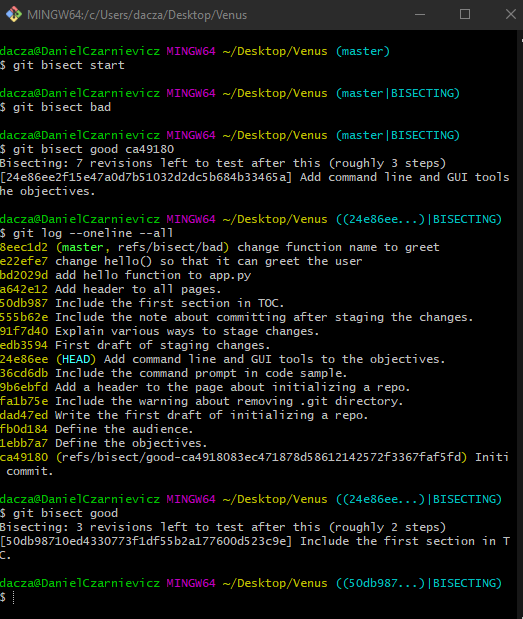
If we now run git log --oneline --all we see that master is at the top and it's referenced as a bad commit. At the bottom of the log, we see that there's a second reference for our bad commit. Our HEAD is at commit 24e86ee. This means that our working directory has been restored to this point in time. Therefore, we can test and see if the bug is here already. If it is, that means that the bug was introduced somewhere between ca49180 and HEAD. If it is not, then the bug was introduced somewhere between HEAD and master. Either way, we eliminated half of all possible commits in one try.
For this demo, let's tell Git that this is a good commit
git bisect good
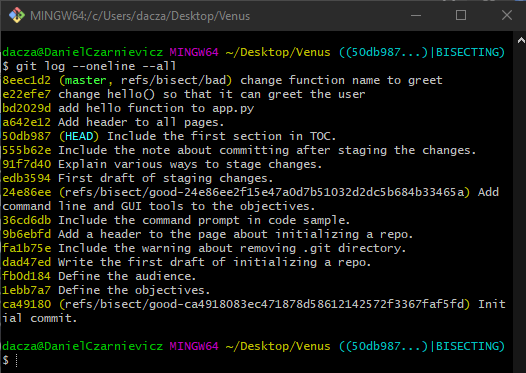
Now Git is telling us that there are 3 revisions left and that it will take 2 steps to inspect them. Our HEAD has now moved to commit 50db987. If we look at the log again, we see that it has moved up. Our repository has once again been checked out to this commit. So we can test our application here and see if the bug is there or not. Again for this demo, we'll tell Git that this is a good commit. So again we run
git bisect good
And we'll do it one more time so that it moves to the commit prior to master.
Now we are at commit e22efe7 and suppose that we found the bug here. We'll tell Git that this is a bad commit
git bisect bad
Now Git knows which one was the first bad commit and it's giving us all the information about that commit: author, date, a summary of changes.
Now we can inspect and test our code here and find the bug. Once we know how to fix it, we can move our HEAD back to master and implement the fix.
git bisect reset
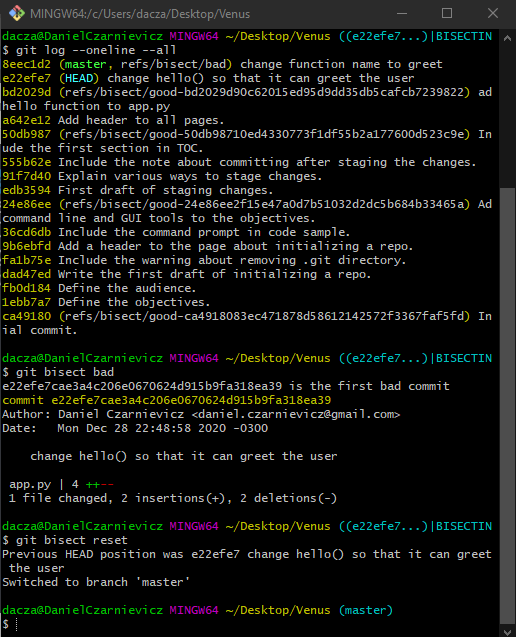