Sometimes we don't want Git to track certain files. To handle this we use a special file "called" .gitignore. This file has no name, only an extension. We should place it at the root of our project. To create it we run
touch .gitignore
If at the time of creating it we already have a file or sub-directory that we want to add to it, we can use
echo file_name.ext > .gitignore
If we already have a .gitignore file and we want to add a new file to it (i.e. we want to append the .gitignore file), we run
echo new_file_name.ext >> .gitignore
If we want to ignore an entire sub-directory, we run
echo sub-dir_name/ >> .gitignore
Once we've created our .gitignore file, we can inspect it with VS Code by running
code .gitignore
As an example, let's create a logs sub-directory and add it to our .gitignore file. First, we create the sub-dir and a file inside that sub-dir with
mkdir logs && echo hello > logs/dev.log
Next, we add it to (and create) the .gitignore file by running
echo logs/ > .gitignore
Now, if we run a git status, we'll see that the .gitignore file is only in the Working Directory, so we'll
and
to commit it to our Repository. But notice that we didn't stage nor committed our logs sub-dir.
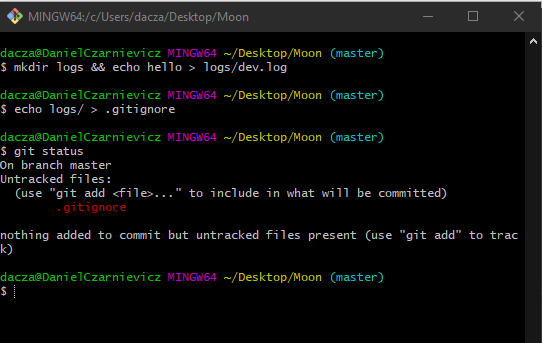
Inside our .gitignore we can have as many items as we want. We can add new files as file_name.ext, we can add entire sub-directories as sub-dir_name/, or we can use patterns. So, for example, adding config.py will cause Git to ignore the file named config.py. Adding *.js will cause Git to ignore all files with a .js extension in our project. Adding logs/*.log will cause Git to ignore all files with extension .log in the logs/ sub-dir, but not other files in that sub-dir, nor files with the .log extension in other directories inside our project.
Keep in mind that all of this only works if you haven't added a file to the repository already. If you have, Git will keep on tracking it. If you added a file to the repository and you now want Git to stop tracking it, you need to remove it from the staging area. To do this we'll use
git rm --cached -r file_name
Now you can commit this deletion and Git will stop tracking the file. Do not forget the --cached option or Git will remove the file both from the staging area and the working directory!!